An SAP system can perform FTP data transfer directly in ABAP. The function module to open the FTP connection is FTP_CONNECT. Password handling however is tricky. Please also check whether you could use an SAP PO system for your data transfer.
Think about the alternative of a PO system first!
While you can do FTP transfers directly in ABAP please check the alternative of using an SAP PO system first. SAP PO is a system designed for data transfers in many protocols, FTP included and many companies already have an accompanying PO system in use.
The big advantage of using a PO system for data transfer is centralized logging and centralized error handling, including restarting FTP transfers after an error.
So let us assume that you did check this alternative and that you came to the conclusion that you still need to do FTP directly in ABAP. Here is what you have to do:
Allow connections to your FTP server
SAP has an extra security layer for FTP connections. You can only initiate connections to those servers that are listed in table SAPFTP_SERVERS. So before you can talk to an FTP server you have to edit this table, either by SM30 or by direct table access like SE16N or whatever direct table access is permitted in your company.
This is how the table SAPFTP_SERVERS looks like:
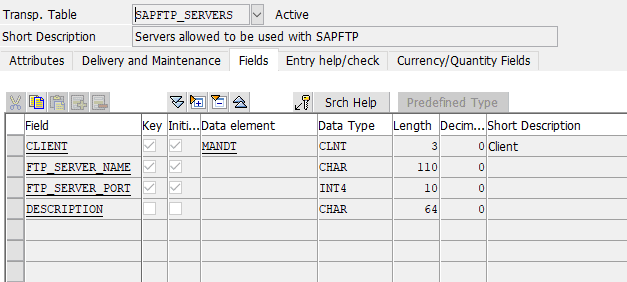
So you have to enter
- a server name
- a port, usually this will be port 21
- a description
Scramble your password.
The odd (and tricky) part of doing an FTP transfer directly from ABAP is scrambling your password.
The actual ftp transfer is handled by a program on the application server provided by SAP. This program expects a coded password, probably for security reasons. It does not really make sense, because it assumes that the application server might be compromised. But if it is, then what else is also compromised. Nevertheless you have to scramle the password.
You can use the function module HTTP_SCRAMBLE to do this. The input is your password. You also have to pass the password length separately. The output is the scrambled password.
The key to use for scrambling is ‘26101957’. This key is fixed, it is exactly the string that the command line program uses to decode the password.
lv_len = strlen( pa_pwd ). call function 'HTTP_SCRAMBLE' exporting source = pa_pwd sourcelen = lv_len key = '26101957' importing destination = pa_pwd.
Use ftp_connect to open the ftp connection
Now you are ready to open the ftp connection. You use ftp_connect.
You need:
- username
- (scrambled) password
- server name (remember that you need to have this server in table SAPFTP_SERVERS)
- and an RFC destination
The RFC destination to use is ‘SAPFTPA’. This starts an ftp connection to on the application server. It is also possible to start the ftp connection in the SAP GUI from your Windows PC. This would not work in batch processing and generally, for security reasons, this would be a bad idea to have your Windows PC talk with an FTP server on the other side of this planet. So I will not tell you which RFC destination you would have to use to do this.
What you get back is a handle, an integer code in an internal table of active ftp connections.
call function 'FTP_CONNECT' exporting user = pa_user password = pa_pwd * ACCOUNT = host = pa_srvr rfc_destination = 'SAPFTPA' * GATEWAY_USER = * GATEWAY_PASSWORD = * GATEWAY_HOST = importing handle = lf_handle exceptions not_connected = 1 others = 2. if sy-subrc <> 0. write: / 'abort in FTP_CONNECT with subrc=', sy-subrc. exit. endif.
Write your data into your ftp connection
Now you are ready to write your data into the ftp connection. Use function module FTP_R3_TO_SERVER to do this. SAP expects your data in the form of text lines. At least that is what this example uses. The function module also has a parameter “blob” for binary data that I have not used so far.
call function 'FTP_R3_TO_SERVER' exporting handle = lf_handle fname = pa_path character_mode = 'X' tables text = lt_text exceptions tcpip_error = 1 command_error = 2 data_error = 3 others = 4. if sy-subrc <> 0. write: / 'abort in FTP_R3_TO_SERVER with subrc=', sy-subrc. exit. endif.
Don’t forget to disconnect
Finally don’t forget to call FTP_DISCONNECT in order to close your FTP connection.
call function 'FTP_DISCONNECT' exporting handle = lf_handle.
Putting it all together
Here is the complete ABAP code:
report zftp_transfer. parameters: pa_srvr type char234 obligatory, pa_user type char234, pa_pwd type char234, pa_path type char234 obligatory, pa_cont type char234 obligatory. start-of-selection. data: lf_handle type i, lv_len type i, lt_text type table of char8000. write: / 'server', pa_srvr. write: / 'user', pa_user. write: / 'password', pa_pwd. write: / 'path', pa_path. write: / 'content', pa_cont. exit. lv_len = strlen( pa_pwd ). call function 'HTTP_SCRAMBLE' exporting source = pa_pwd sourcelen = lv_len key = '26101957' importing destination = pa_pwd. write: / 'scrambled password', pa_pwd. call function 'FTP_CONNECT' exporting user = pa_user password = pa_pwd * ACCOUNT = host = pa_srvr rfc_destination = 'SAPFTPA' * GATEWAY_USER = * GATEWAY_PASSWORD = * GATEWAY_HOST = importing handle = lf_handle exceptions not_connected = 1 others = 2. if sy-subrc <> 0. write: / 'abort in FTP_CONNECT with subrc=', sy-subrc. exit. endif. " transfer content to string array append pa_cont to lt_text. call function 'FTP_R3_TO_SERVER' exporting handle = lf_handle fname = pa_path character_mode = 'X' tables text = lt_text exceptions tcpip_error = 1 command_error = 2 data_error = 3 others = 4. if sy-subrc <> 0. write: / 'abort in FTP_R3_TO_SERVER with subrc=', sy-subrc. exit. endif. call function 'FTP_DISCONNECT' exporting handle = lf_handle.
A final word of caution
This approach does work, but nevertheless it would be better to use your SAP PO system for FTP transfers. You get logging for free, you get monitoring for free, customization options are a lot better, you have build-in support for http proxies etc.
Direct FTP transfer in ABAP should be a rare exception only.
You can find more articles about SAP here.